Arduino User Input Devices
Lesson 1
Button
This is the first lesson in the Arduino User Input devices. You will learn which devices work as inputs for the Arduino, how to connect and use them.
The Button
A button is a simple on-off switch. There are many kinds of buttons, distinguished by the mechanism used to close or open a circuit, but essentially all buttons belong to one of two families: those that keep the connection in either an open or a closed state, and those that return to their original (default) state.
Here are some examples of switches.
Clock-wise from the top:
- A toggle switch. It stays open or closed after pushing it.
- An on/off switch that also remains open or closed.
- A momentary button that remains closed while pressure is applied to it, then returns to the open position.
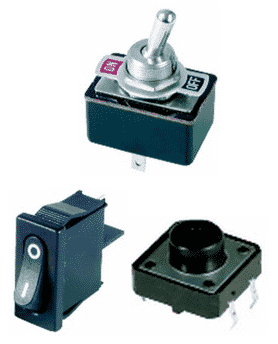
Button types
A keyboard key or a door bell button are both momentary buttons. Momentary buttons are also known as "biased" because they have a tendency to return to their original position. A light switch, on the other hand, stays at the position it was pushed to, so it is often called "un-biased".
Experiment
Let's create a simple circuit to demonstrate a button.
We don't really need the Arduino for this. A battery, an LED, a resistor and the button itself would suffice.
But, using the Arduino is simple enough, and it's already got an LED in pin 13 we can use anyway, and no need to worry about a battery pack.
Plus, we can use the monitor to actually see a message when the button is pressed.
So, we'll setup this circuit (below).
Once assembled, the project will look like this (below as well):
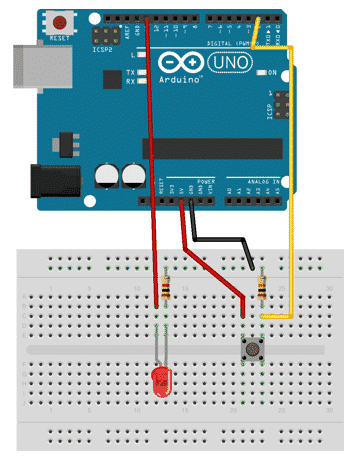
Circuit schematic
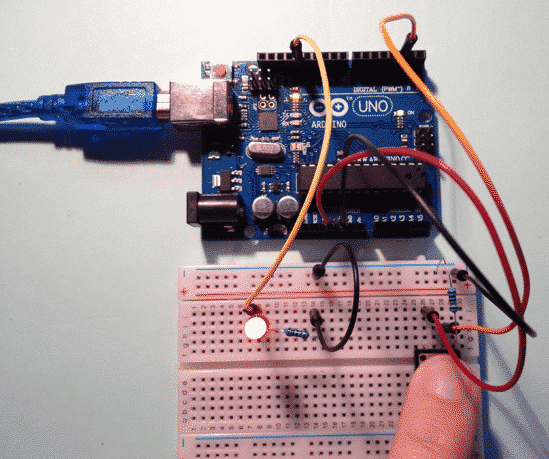
Our circuit in action!
Sketch
The sketch is simply taking a reading from digital pin 2, where one of the pins of the button is connected, and writing a value to digital pin 13, where the LED is connected.
I could have written this script to be even smaller, but I will leave that for you to do as an exercise.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
/* Pushbutton sketch a switch connected to pin 2 lights the LED on pin 13 */ const int ledPin = 13; const int inputPin = 2; void setup() { pinMode(ledPin, OUTPUT); // choose the pin for the LED // choose the input pin (for a // pushbutton) // declare LED as output // declare pushbutton as input pinMode(inputPin, INPUT); } void loop(){ int val = digitalRead(inputPin); // read input value if (val == HIGH) { digitalWrite(ledPin,HIGH); } else { digitalWrite(ledPin,LOW); } } |
And that's how you can use a momentary button with the Arduino!
"Arduino User Input Devices" series
Ready for some serious Arduino learning?
Start right now with Arduino Step by Step Getting Started
This is our most popular Arduino course, packed with high-quality video, mini-projects, and everything you need to learn Arduino from the ground up.
Jump to another article in this series.
Last Updated 1 year ago.
We publish fresh content each week. Read how-to's on Arduino, ESP32, KiCad, Node-RED, drones and more. Listen to interviews. Learn about new tech with our comprehensive reviews. Get discount offers for our courses and books. Interact with our community. One email per week, no spam; unsubscribe at any time