Arduino programming guide series
Boolean arrays
It is a common practice to use arrays to store chars, ints, or double values.
But arrays can also store booleans. Here's how.
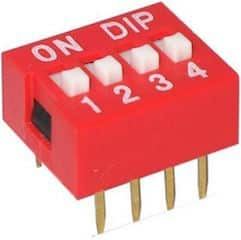
A boolean data-type is one that can take only two possible values. Usually these values are depicted as "TRUE" or "FALSE". You can also see them as "ON" or "OFF", or "1" or "0".
You may intuitively think that a boolean data-type can be stored with a single bit, instead of a full byte. Let's correct this right now: A boolean data type may be one of two possible values, but it still takes a full byte to store it in SRAM.
Still, even though boolean data-types will not save an SRAM, they are better suited than alternatives, like int or byte in many applications.
Let's look at an example.
Imagine that you have a set of dip switches connected to the digital pins of an Arduino.
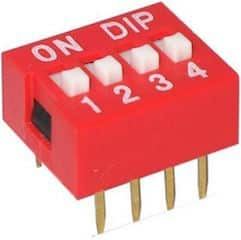
Let's say that your dip switches are configured to produce the code "0101" in digital pins 3, 4, 5, 6.
You can capture this code and store it into a boolean array like this:
boolean dip_switch[4];
dip_switch[0] = digitalRead(3); // a boolean data type
dip_switch[1] = digitalRead(4); // a boolean data type
dip_switch[2] = digitalRead(5); // a boolean data type
dip_switch[3] = digitalRead(6); // a boolean data type
The configuration of the DIP switches is now stored in an array of type "boolean".
Boolean is a non-standard data type defines in the Arduino language, that is identical to the bool data type. In this example code, you could substitute "boolean" for "bool" without changing the outcome. In fact, the Arduino documentation recommends that you use "bool" instead of "boolean".
If you want to treat the dip_switch array as a half-byte word, then you can convert it to a decimal. You can do this with this code:
byte encodebool(boolean* arr)
{
byte val = 0;
for (int i = 0; i<4; i++)
{
val << = = 1;
if (arr[i]) val | = 1;
}
return val;
}
If dip_switch contains "0,1,0,1", then encodebool(dip_switch) will return "5".
With this simple example, you can use a DIP switch, store the positions of its switches to a boolean array, and convert the half-byte set on the DIP switch to a decimal on demand.
New to the Arduino?
Arduino Step by Step Getting Started is our most popular course for beginners.
This course is packed with high-quality video, mini-projects, and everything you need to learn Arduino from the ground up. We'll help you get started and at every step with top-notch instruction and our super-helpful course discussion space.
Jump to another article
3. Focus on the type parameter in "println()"
4. "0" or "A0" when used with analogRead()?
5. What is the "_t" in "uint8_t"?
6. Save SRAM with the F() macro
7. What is the gibberish in the Telnet output?
9. Confusing keywords? follow the source code trail
10. The interrupt service routine and volatile variables
11. The problem with delay() and how to fix it
12. How to deal with the millis rollover
13. Can you use delay() inside Interrupt Service Routine?
15. A closer look at line feeds and carriage returns
16. Understanding references and pointers
17. Simple multitasking on the Arduino
19. Concurrency with the Scheduler library on the Arduino Due and Zero
20. Bitshift and bitwise OR operators
21. What is a "static" variable and how to use it
22. Understanding the volatile modifier
Last Updated 1 year ago.
We publish fresh content each week. Read how-to's on Arduino, ESP32, KiCad, Node-RED, drones and more. Listen to interviews. Learn about new tech with our comprehensive reviews. Get discount offers for our courses and books. Interact with our community. One email per week, no spam; unsubscribe at any time