MICROPYTHON WITH THE ESP32 GUIDE SERIES
MicroPython Programming With Files
In this lesson I will show you how to split your MicroPython programs into multiple files so that you can better organize your code.
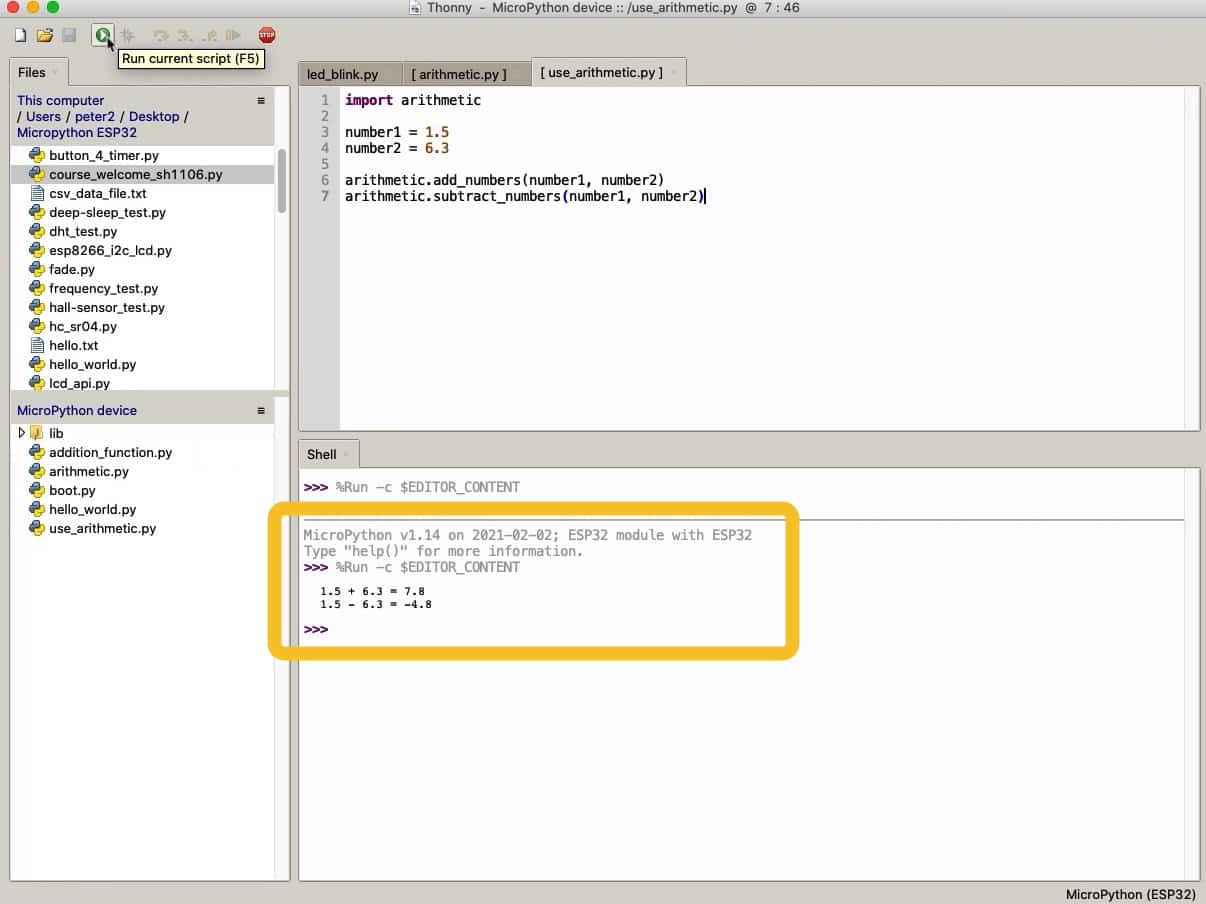
In this lesson I will show you how to split your MicroPython programs into multiple files so that you can better organize your code.
Doing so is particularly useful as your programs become larger and more complicated.
One program, two files
To show you how to split your MicroPython program into multiple files, I have prepared a simple example. The example contains two files, that I list below (first, screenshots that show how these files look in Thonny, followed by the code):
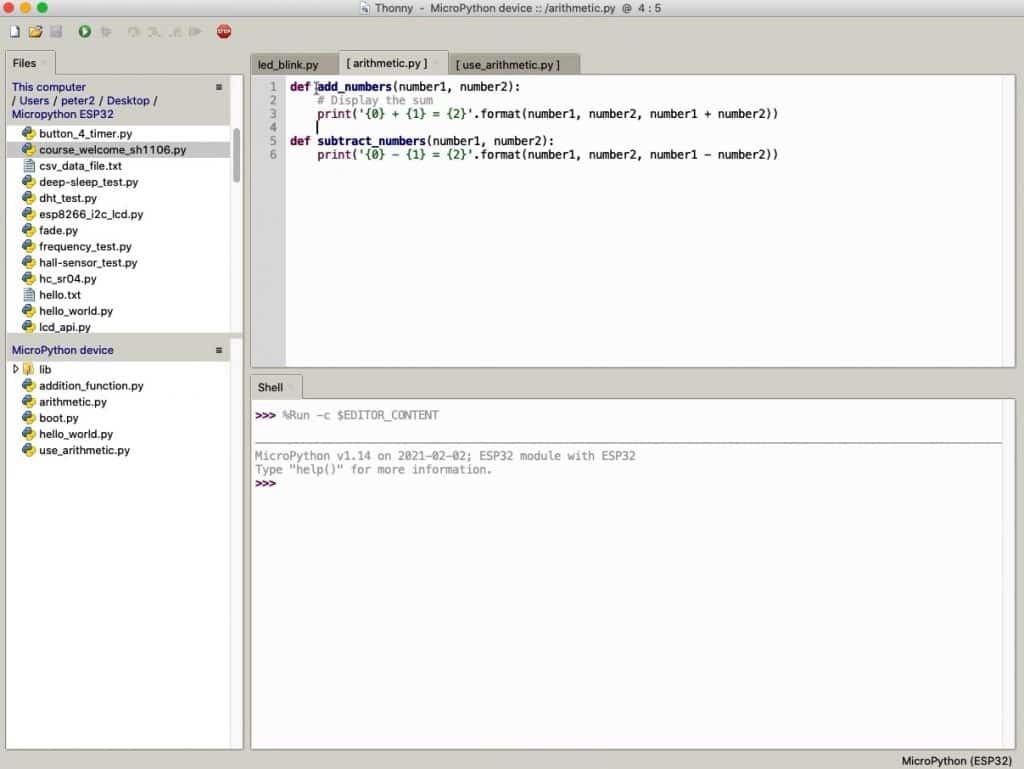
arithmetic.py in a Thonny tab.
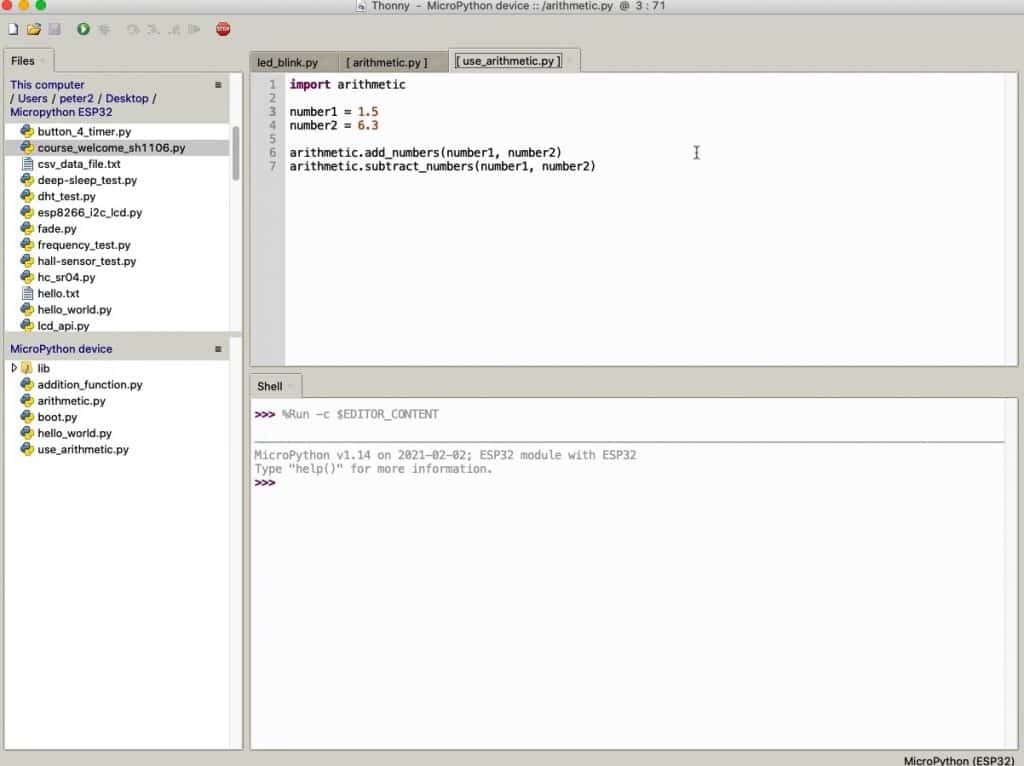
use_arithmetic.py in a Thonny tab.
arithmetic.py
def add_numbers(number1, number2):
# Display the sum
print('{0} + {1} = {2}'.format(number1, number2, number1 + number2))
def subtract_numbers(number1, number2):
print('{0} - {1} = {2}'.format(number1, number2, number1 - number2))
use_arithmetic.py
import arithmetic
number1 = 1.5
number2 = 6.3
arithmetic.add_numbers(number1, number2)
arithmetic.subtract_numbers(number1, number2)
arithmetic.py
The first file is titled "arithmetic.py", and it contains two functions.
The first one is "add_numbers" that receives two numerical parameters, adds them, and prints the result to the shell.
The second function is titled "subtract_numbers". This function also receives two parameters, subtracts them and prints out the result to the shell.
Don't worry about the details of how these programs work. I have a complete section in the course dedicated to the MicroPython language where I explain everything, including the use of the format function, how to create custom functions, parameters and classes.
use_arithmetic.py
The second file is titled "use_arithmetic.py".
In the first line, we use the import keyword to import the contents of the "arithmetic.py" file. With this import, we can now use the two functions defined inside arithmetic.py without having to write them again.
In other words, the file arithmetic.py consists of a small MicroPython module that we can import and use in other programs.
To import a module, we use the import keyword followed by the name of the file that contains the code that we want to import, without the ".py" extension.
Next in use_arithmetic.pu, I create two variables to hold the numbers that I want to use later, and give each an initial value.
Finally, I call the calculation functions that exist in the arithmetic module to do the addition and subtraction between those numbers.
Execute the program
As with any MicroPython program in Thonny, to run it, simply click on the play button.
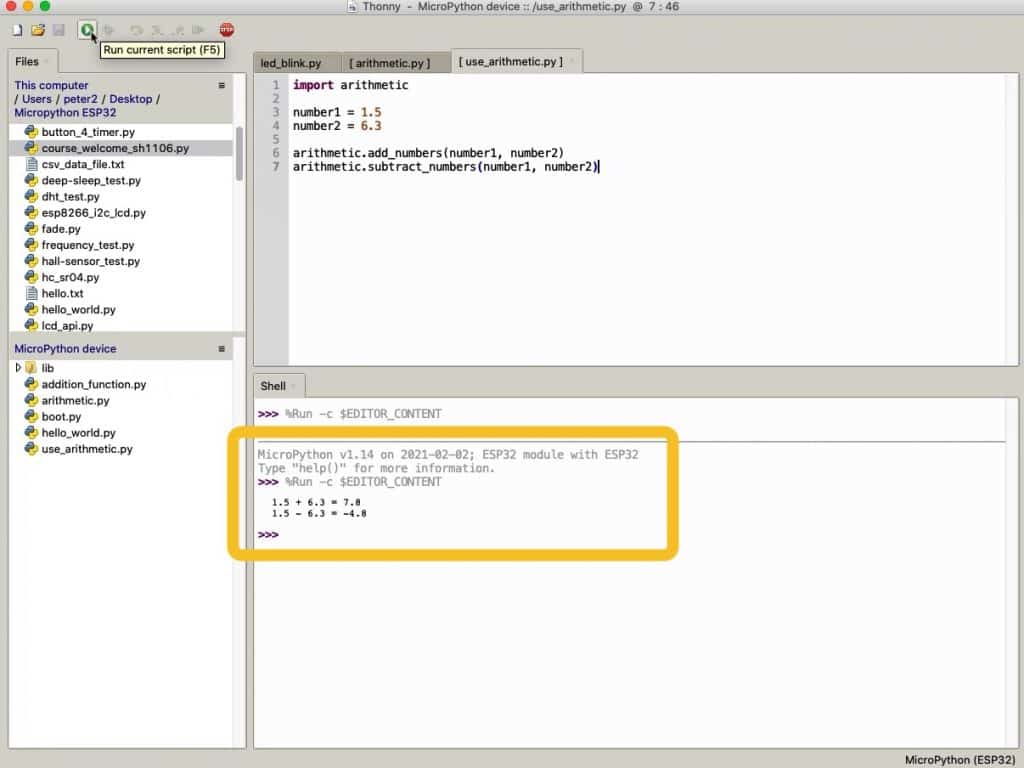
My modular program has executed. Output is in the shell.
Why split a program?
The files in this example are tiny. But imagine your programs getting bigger and bigger as you become more proficient in the language. Being able to split them into multiple files results in programs that are more efficient, easier to manage, easier to modify and easier to share. Each file can be dedicated to a single task or a set of closely related tasks.
Learn MicroPython for the ESP32
With this video course, you will learn how to use the
MicroPython programming language with the ESP32 micro-controller.
MicroPython is the perfect language for anyone looking for the easiest (yet still powerful) way to program a micro-controller.
Jump to another article
1. What is MicroPython?
2. MicroPython vs Python
3. MicroPython resources
4. MicroPython compatible boards
5. Getting started with Thonny IDE
6. How to install the MicroPython firmware
7. Setup an interpreter in Thonny IDE
8. How to write and execute a MicroPython program
9. Thonny IDE with the Raspberry Pi Pico
10. Thonny IDE with the BBC Micro:bit
11. Thonny IDE advanced configuration
12. How to find MicroPython packages at PyPi
13. The MicroPython shell
14. MicroPython Programming with files
15. How to interrupt a running program
16. How to run a program at boot
17. How to debug MicroPython program
Last Updated 1 year ago.
We publish fresh content each week. Read how-to's on Arduino, ESP32, KiCad, Node-RED, drones and more. Listen to interviews. Learn about new tech with our comprehensive reviews. Get discount offers for our courses and books. Interact with our community. One email per week, no spam; unsubscribe at any time