Arduino programming guide series
The interrupt service routine and volatile variables
Interrupt service routines (ISR) must be as small as possible. If your sketch has to do time-consuming work after an interrupt event, you can use volatile variables to capture data and process it outside of the ISR.
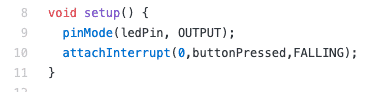
Last Updated 1 year ago.
We publish fresh content each week. Read how-to's on Arduino, ESP32, KiCad, Node-RED, drones and more. Listen to interviews. Learn about new tech with our comprehensive reviews. Get discount offers for our courses and books. Interact with our community. One email per week, no spam; unsubscribe at any time