MICROPYTHON WITH THE ESP32 GUIDE SERIES
Introduction To MicroPython With The ESP32: What Is MicroPython?
The Guides in this series are dedicated to MicroPython for the ESP32. In this first lesson, I will introduce you to MicroPython, its reason to exist, how it relates to Python, and its most important characteristics.
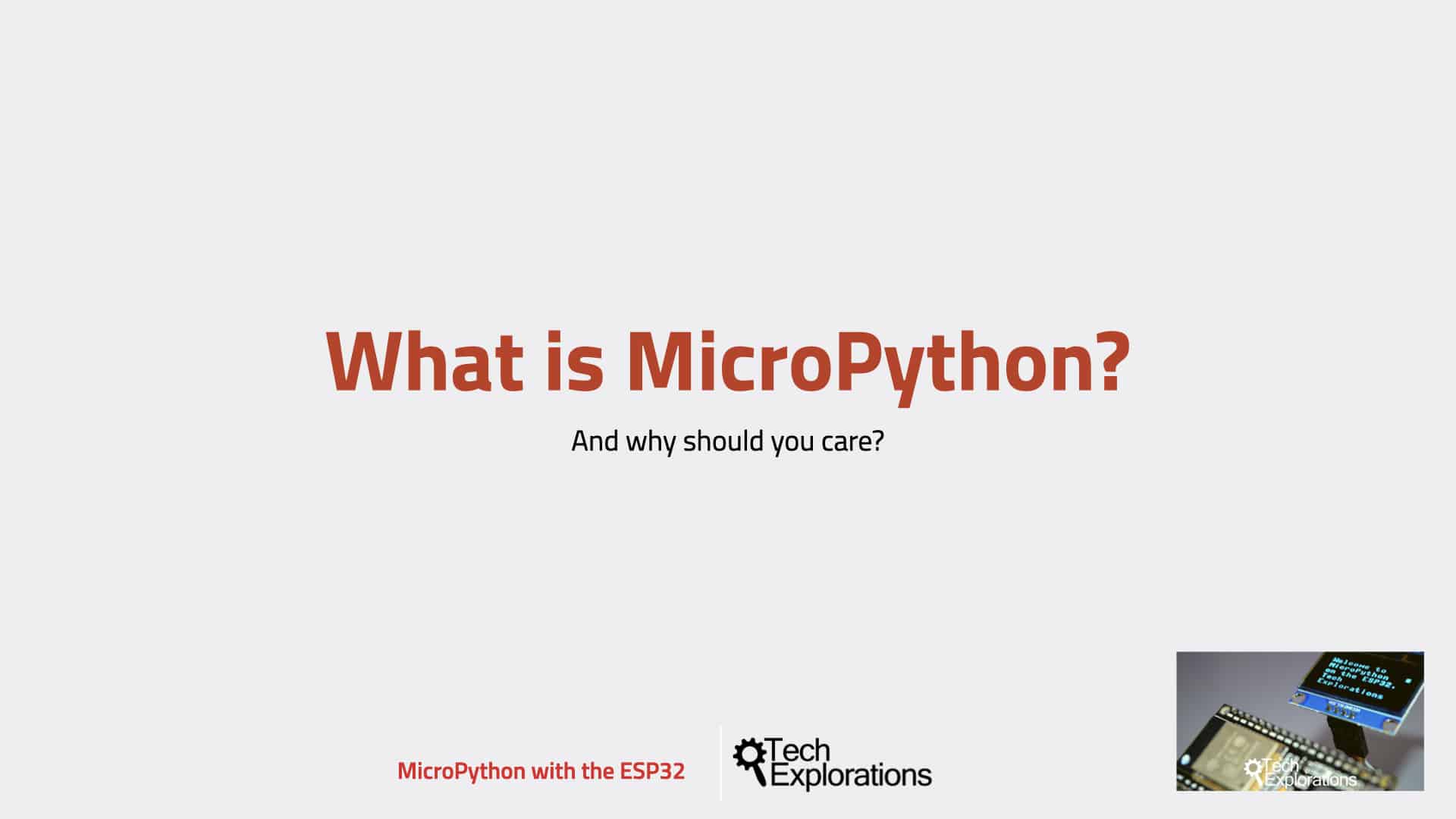
Around mid-2014, Damien George published a new programming language for microcontrollers, called MicroPython. This publication was a successful completion of an ambitious Kickstarter project that begun in 2013.
At the time, microcontroller programming was dominated by the C language.
If you are familiar with the Arduino, you know what C looks like. On a microcontroller like the Arduino, C is not very difficult to learn, however things do get more complicated as programs get bigger.
As microcontrollers started becoming more powerful, more people started being interested in programming them. Many of them were first-time programmers. This included people in all age brackets.
Damien wanted to create a language that would work on microcontrollers that would be much easier to learn and use than C. He did not want to reinvent the wheel, so he chose Python as his prototype.
His challenge was to create a language that can mimic Python that can run on the bare metal of a microcontroller, without an operating system.
So, he created MicroPython.
What is MicroPython?
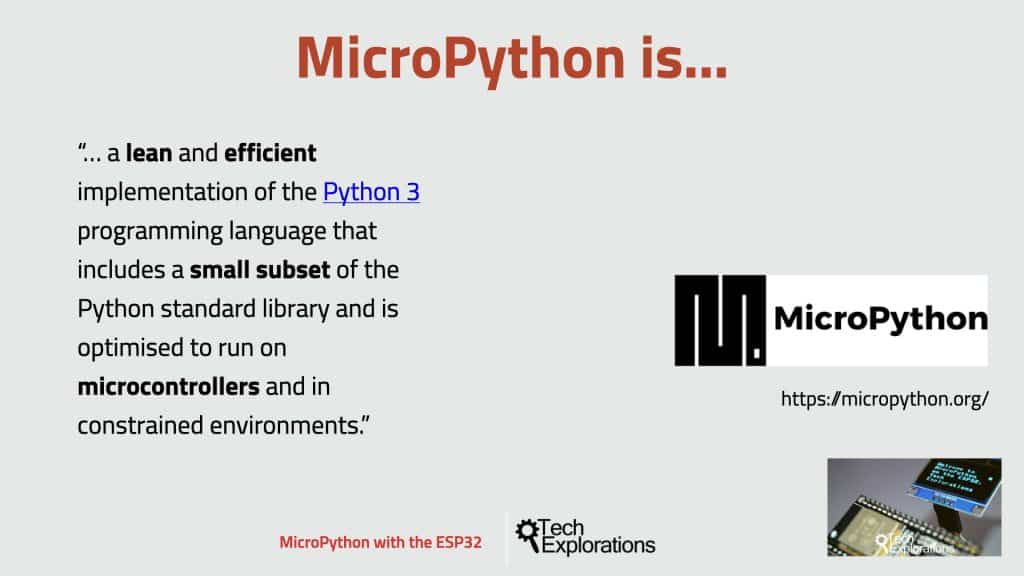
Here is a description of the language from the MicroPython website (emphasis in bold is mine):
“MicroPython is a lean and efficient implementation of the Python 3 programming language that includes a small subset of the Python standard library and is optimised to run on microcontrollers and in constrained environments.”
Python ≠ MicroPython
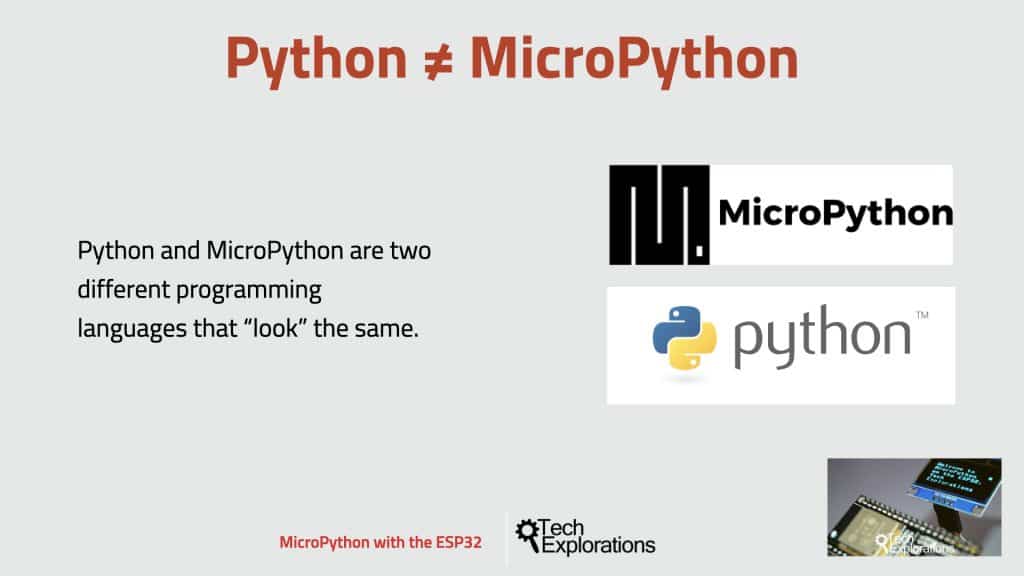
Because MicroPython contains the word “Python”, it is easy to become confused and think that MicroPython is simply a smaller version of Python.
It is the same confusion that I have seen in the past between Java and Javascript.
While Python and MicroPython have a similar name, they are totally different languages, with a different set of goals and implementation.
I talk more about the differences between Python and MicroPython in a later lecture. For now, I just want to make sure that you are not confused by the similarity in the name.
Excellent for learning and using
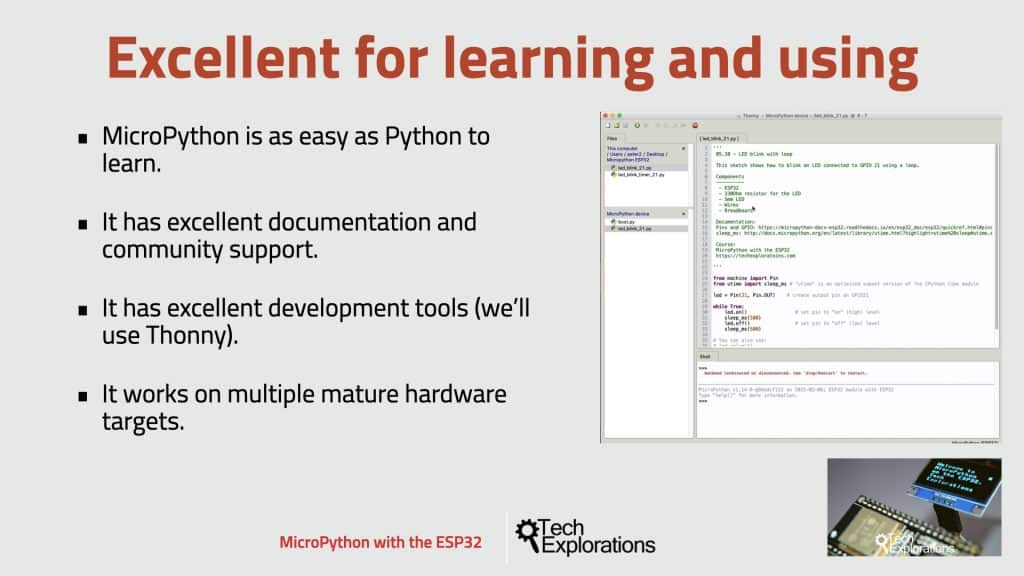
What MicroPython has taken from Python is the language architecture, its programming philosophy for code readability, and a huge pool of programmers that already know how to use Python.
Pythonistas can quickly become MicroPythonistas and write programs for microcontrollers.
According to the “PYPL PopularitY of Programming Language Index”, Python is the most popular programming language in the world, with a 30% share. This index is calculated based on the amount of searching is done on Google for programming language tutorials or resources.
As a comparison, C/C++ used by the Arduino boards ranks 5th place in this index.
This popularity translates to a Python universe that is filled with all the documentation, libraries and community support you will ever need.
MicroPython is as easy as Python to learn, and follows Python’s tradition for excellent development tools and documentation. In this course, you will see me constantly browsing through the MicroPython core documentation, as well as many of the excellent libraries we’ll be using.
In terms of tools, you have many choices. In this course, I’ll be using Thonny. But, you can also choose tools such as upycraft, and the Mu editor.
What I really like about Thonny is that is a full Python editor on its own merit, with excellent debugging tools, but also fully supports MicroPython on the ESP32 as well as other target boards like the Raspberry Pi Pico and the BBC Microbit.
Another big advantage of MicroPython is that once you learn it, you can use your skills across multiple hardware targets. At the time I am recording this lecture, MicroPython has support for the original Myboard v1 and D-series, as well as third-party boards such as the STM32 Nucleo and Discovery boards, the Espruino Pico, the Raspberry Pi Pico, the WiPy, the ESP8266 and ESP32, the TinyPico, and the BBC Micro:Bit.
This was just a partial list.
MicroPython features
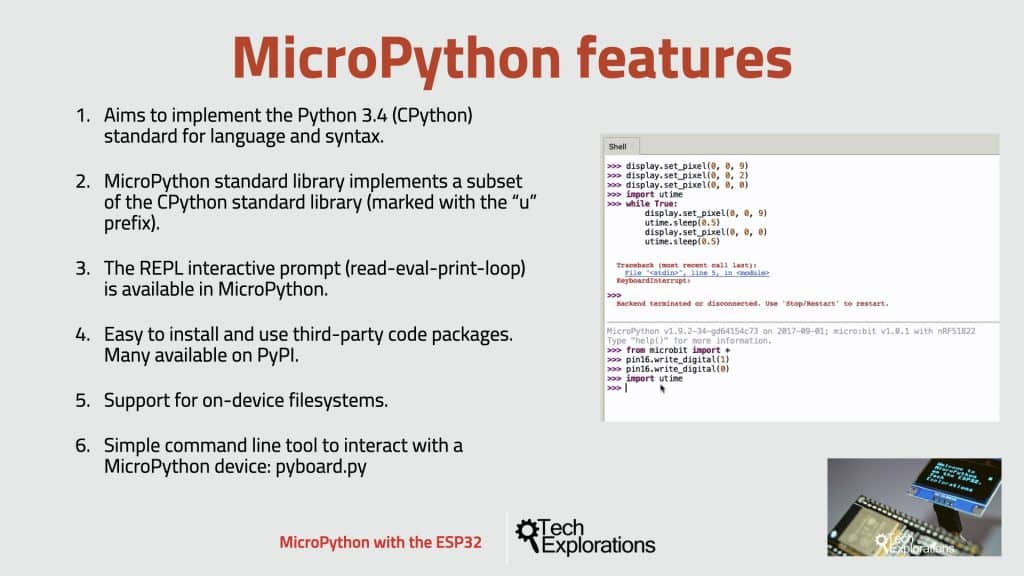
Let’s take a quick tour of MicroPython’s most important features.
Implements Python 3.4 language and syntax
First and most important for anyone new to this language, is that MicroPython aims to implement the Python 3.4 standard for language and syntax.
This simply means that anyone who already programs in Python 3.4 will be able to start programming in MicroPython immediately. Python 3.4 reserved keywords, operators, functions, even the infamous whitespace indentation is faithfully implemented in MicroPython.
uPython standard library is a subset of CPython standard library
Because MicroPython targets embedded computers and microcontrollers, it is not possible to implement the full Python standard library, with all of its modules and methods.
There’s simply not enough storage on the target devices.
Therefore, MicroPython implements a selected subset of CPython’s standard library. Even that, is implemented with emphasis in efficiency. MicroPython versions of Python libraries have a name with the “u” letter prefix.
uPython has a REPL
MicroPython has an Interactive Interpreter mode, also known as “REPL”. REPL stands for “Read-Eval-Print-Loop”. Think of it as a command line for Python. You can use this command line to issue Python instructions or even code blocks. The REPL will evaluate this Python code immediately.
The MicroPython REPL is fully featured, with auto-indent, auto-completion, ability to interrupt a running program with Ctrl-C and invoke a soft reset.
There is also a paste mode, and you can use the special “_” underscore variable that stores the output of the previous computation. I this course, I’ll be using the REPL extensively to demonstrate and test code.
Easy to install 3rd-party packages
Outside of the MicroPython standard library, there are countless libraries contributed by users and published online on repositories like Github and PyPI (the Python Package Index).
Similar to CPython, MicroPython has a simple mechanism for including external code to your programs. In this course, I’ll show you how to find and use external libraries that make it easy to integrate hardware components like screen and sensors to your MicroPython projects.
Supports on-device filesystems
MicroPython has the ability to access a small filesystem on the target microctroller device. This filesystem makes it possible to store your MicroPython programs, supporting library files, and arbitrary files such as text files for storing sensor data or credentials for networks and IoT services, or bitmap image files.
In this course, I have prepared several examples where I demonstrate how to use the file system on the ESP32.
Simple command-line tool
MicroPython has a single command-line Python tool that allows you to run a script or access the filesystem on a target device.
This tool is called pyboard.py.
In this course, we will not be using this tool because Thonny IDE has build-in support for MicroPython on a variety of target devices, including the ESP32. However, I mention pyboard.py here because it provides a simple way to interact with the REPL, run programs, and upload or download files from the device file system.
MicroPython on different devices
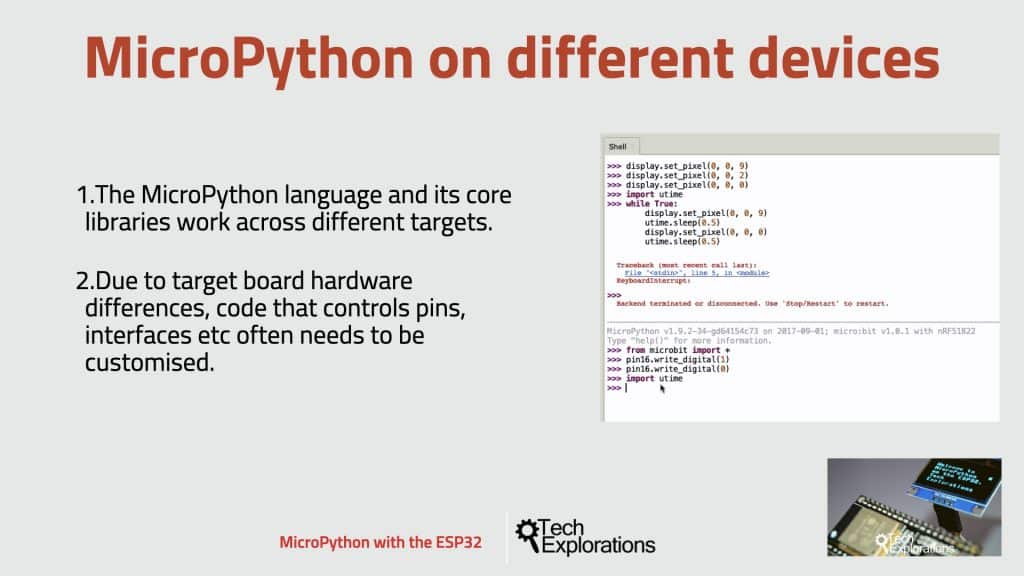
MicroPython works on many different microcontrollers.
The diversity of the hardware means that not all MicroPython code will work across those devices without modifications.
In general, there are two points to remember in relation to sharing MicroPython code across different targets.
uPython language and core libraries work across different targets
Most of the code that uses MicroPython standard library functions and the core of the language will work without modifications.
Language syntax, reserved keywords, control structures, and functions that come from standard libraries like math (for mathematics), uos (for basic operating system services) and utime (for time and date related functions), will work across all MicroPython hardware targets.
Code that controls pins, interfaces needs customisation
On the other hand, any functionality that is uniquely implemented on a microcontroller requires a unique implementation in MicroPython.
For example, the way that digital pin functionality is implemented between the ESP32 and the Raspberry Pi Pico differs.
It is it a similar case to how functions relating to network, the I2C and SPI interfaces, and the analog to digital converters are implemented across boards. These differences are reflected in the MicroPython implementation for each board.
For this reason, in addition to the standard library, MicroPython has libraries specifically implemented for each supported board. You should take a bit of time to study your target device special libraries so that you know what is available and how you can go about taking advantage of the device capabilities.
uPython may not access all hardware features
One more thing: Not al device capabilities can be access through MicroPython. For example, in the ESP32, there is no MicroPython module for Bluetooth, although there is for Wifi.
MicroPython is readable
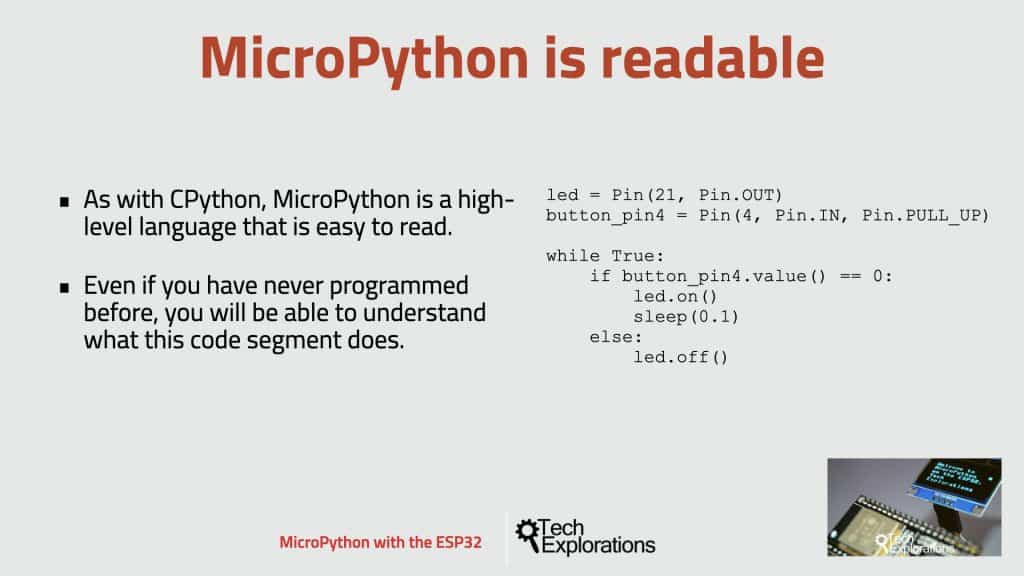
Let’s wrap up this lesson by going back to MicroPython’s most important attribute.
MicroPython, like CPython, is designed to be readable. It almost reads like natural language.
This is an example code segment from one of the lectures in this course. Even if you have never seen MicroPython before, and perhaps have never programmed before, you will be able to make fairly accurate inferences about what this code is supposed to do.
Yes, you do need to have a basic understanding of electronics. Without that, keywords like “Pin.OUT” and “Pin.PULL_UP” will not make sense.
However, the language barrier to entry for MicroPython is minimal. Certainly, it is much lower than the barrier to entry for a language like C or C++.
This is the number 1 reason why Python became so popular, and why MicroPython has been gaining massive support in popularity since the Kick-starter campaign in 2014.
Learn MicroPython for the ESP32
With this video course, you will learn how to use the
MicroPython programming language with the ESP32 micro-controller.
MicroPython is the perfect language for anyone looking for the easiest (yet still powerful) way to program a micro-controller.
Jump to another article
1. What is MicroPython?
2. MicroPython vs Python
3. MicroPython resources
4. MicroPython compatible boards
5. Getting started with Thonny IDE
6. How to install the MicroPython firmware
7. Setup an interpreter in Thonny IDE
8. How to write and execute a MicroPython program
9. Thonny IDE with the Raspberry Pi Pico
10. Thonny IDE with the BBC Micro:bit
11. Thonny IDE advanced configuration
12. How to find MicroPython packages at PyPi
13. The MicroPython shell
14. MicroPython Programming with files
15. How to interrupt a running program
16. How to run a program at boot
17. How to debug MicroPython program
Last Updated 8 months ago.
We publish fresh content each week. Read how-to's on Arduino, ESP32, KiCad, Node-RED, drones and more. Listen to interviews. Learn about new tech with our comprehensive reviews. Get discount offers for our courses and books. Interact with our community. One email per week, no spam; unsubscribe at any time