RASPBERRY PI GETTING STARTED SERIES
A Taste Of Python On The Command Line Interpreter
In this lesson you will practice with the use of the Python language on the Raspberry Pi command like interpreter (CLI).
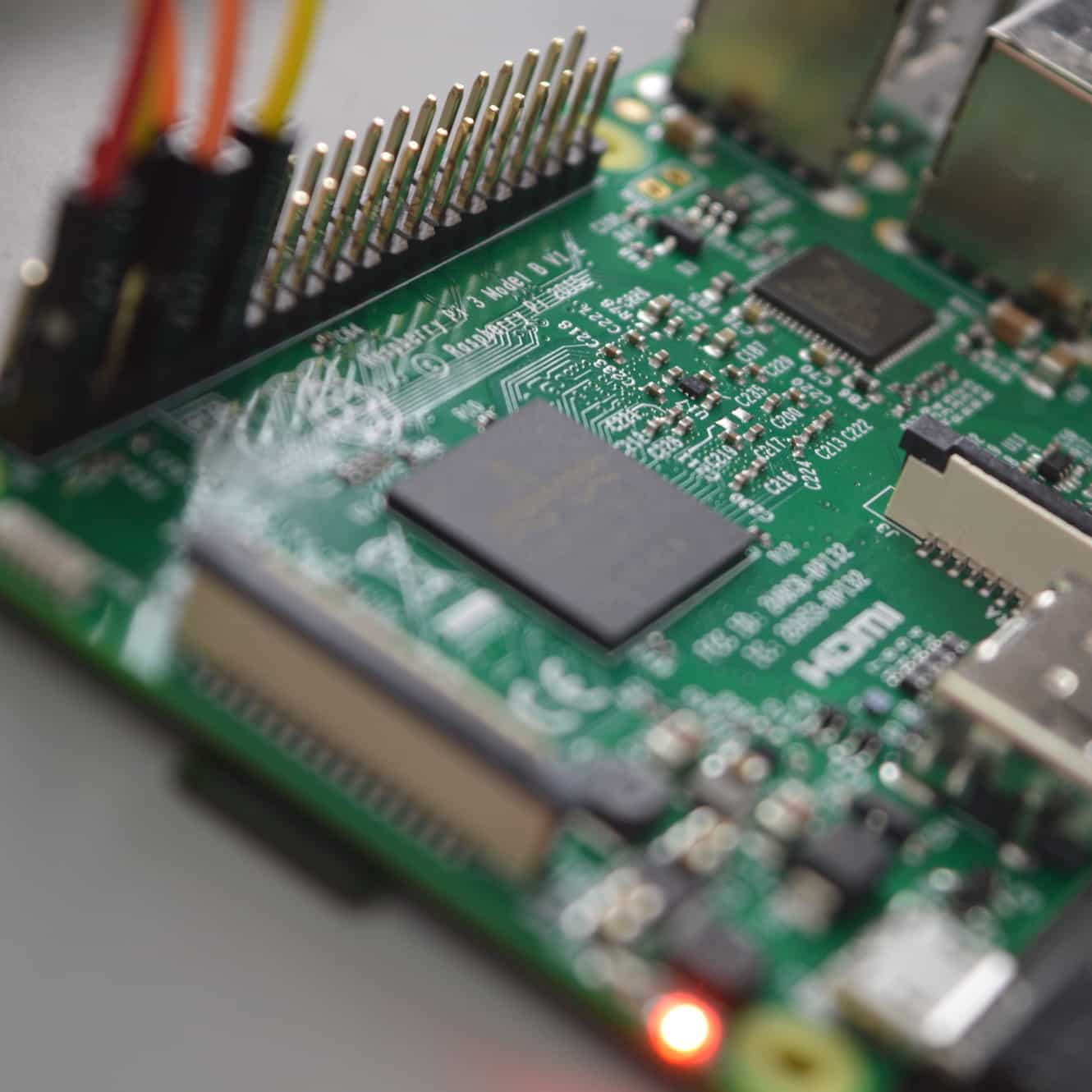
Let’s play with Python!
The Raspberry Pi is a full Linux computer, so you can use virtually any programming language with it. However, Python is the one language that stands out. Python is the language with the widest adoption among Raspberry Pi users. This means that it has (by far) the best and most plentiful documentation, widest range of available libraries that you can use in your programs, and drivers for all sorts of external hardware.
For this reason, we will be using Python in this project.
In this chapter you will try out a few simple experiments with Python that do not require external hardware. This is an opportunity to get used to using Python on the command line.
If you have never worked with Python before, do not worry. We’ll take this one step at a time and gradually build the skills you will need for the project.
Let’s begin.
Log into your Raspberry Pi with the pi user. For me, the login looks like this (in bold is the command that I entered):
Peters-iMac:~ peter$ ssh [email protected]
[email protected]'s password:
Linux raspberrypi-zero.local 4.19.97+ #1294 Thu Jan 30 13:10:54 GMT 2020 armv6l
The programs included with the Debian GNU/Linux system are free software;
the exact distribution terms for each program are described in the
individual files in /usr/share/doc/*/copyright.
Debian GNU/Linux comes with ABSOLUTELY NO WARRANTY, to the extent
permitted by applicable law.
Last login: Tue Mar 24 00:12:09 2020 from 192.168.112.13
SSH is enabled and the default password for the 'pi' user has not been changed.
This is a security risk - please login as the 'pi' user and type 'passwd' to set a new password.
pi@raspberrypi-zero:~ $
At the time I am writing this, Python is available in two versions. Python 2, and Python 3. Python 2 is a legacy version for which support is ending. So, we’ll be working with Python 3.
You can use Python is two “modes”.
First, you can write traditional Python programs and then execute them. We’ll do that very soon.
Second, you can write interactive Python programs in the Command Line Interpreter (CLI). The CLI is a utility that evaluates a Python instruction as you issue it. It is a very good way to quickly try out an idea, or learn how to use a function.
Let’s have a look at using Python on CLI now.
At the command line, type this:
pi@raspberrypi-zero:~ $ python3
Python 3.7.3 (default, Dec 20 2019, 18:57:59)
[GCC 8.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>>
This will invoke the CLI, and present you with the “>>>” prompt. You can type a Python command at the prompt, and the the CLI will evaluate it immediately.
Try out these commands (lines that start with “>>>” are where I have entered a command, lines without the prompt is where Python prints out the result of the command, and the numbers before the “>>>” are there for reference):
1- >>> 1+1
2
2- >>> 17/3
5.666666666666667
3- >>> 17/3 # This is a division
5.666666666666667
4- >>> division_result = 17/3
5- >>> division_result
5.666666666666667
6- >>> print(division_result)
5.666666666666667
7- >>> round(division_result,2)
5.67
8- >>> 'Tech Exporations'
'Tech Exporations'
9- >>> str = 'Tech Exporations'
10- >>> str
'Tech Exporations'
11- >>> print(str)
Tech Exporations
12- >>> print(str + ' ' + 'RPi FS')
Tech Exporations RPi FS
13- >>> 3 * str
'Tech ExporationsTech ExporationsTech Exporations'
14- >>> str[5]
'E'
15- >>> str[5:7]
'Ex'
16- >>> str[0]
'T'
>>>
Let’s take some time to dissect some of these examples.
- In the first two prompts (“1+1” and “17/3”), you did simple arithmetic. You used the “+” and “/“ operators to do addition and division. So, you can use Python as a calculators.
- In the third prompt I have added a comment using the “#” symbol. Any text after the “#” is ignored by Python.
- In the fourth prompt, you created a variable with the name “division_result“ and store the result of the division in it. In Python, variables can store numbers, strings, arrays, and all kinds of objects.
- In the fifth prompt, you typed in the name of the variable only, and what came back from Python is the value stored in it.
- In the sixth prompt you used the “print” function to show the content of the variable. The result is the same as if you have just typed in the name of the variable, and this is specific to how the CLI behaves in these cases.
- In the seventh prompt, you used the “round” function to round the result of the division to two decimal points.
- In prompt 8, you have created a string. Notice that you used single quotes to enclose the string.
- In prompt 9, you stored the string in a variable titled “str”.
- In prompt 10, you retrieved the value stored in “str”.
- In prompt 11, you did the same by using the “print” function.
- In prompt 12, you used the “+” operator to concatenate three smaller strings into a large one. The “+” operator concatenates strings when it is used with strings, and adds numbers when it is used with numbers.
- In prompt 13, you used the “*” operator to multiply a string by a number. The result is the the same string came out multiple times (3 times, in this example).
- In prompts 14, 15, and 16, you use the string as an array of characters. Inside the square brackets, you make reference to the cell of this array from where you want to extract the character. Inside the “str” variable, you have stored the string “Tech Explorations”. The letter in cell “0” is “T”, and in cell “5” is “E”. You can also specify cell ranges, so the letters in the range from 5 (inclusive) to 7 (not inclusive) are “Ex”.
As you can see, Python, while very powerful, is very intuitive for beginners. You can be productive with it very quickly, without any formal study of the language.
In the next chapter, I will show you the basics of functions. Functions is a programming construct that allows us to bundle functions together, and call them with a single name.
Ready for some serious learning?
Start right now with Raspberry Pi Full Stack - Raspbian
This is our most popular Raspberry Pi course & eBook.
This course is a hands-on project designed to teach you how to build an Internet-of-Things application based on the world’s most popular embedded computer.
You will learn how to build this application from the ground up, and gain experience and knowledge with technologies such as...
Jump to another article
1: What is the Raspberry Pi?
2: Raspberry Pi vs Arduino
3: Raspberry Pi operating systems
4: Headless and graphical (GUI) operating systems
5: How to install Raspbian Lite
6: SSH and headless configuration
7: How to set a host name
8: Booting for the first time
9: How to set a fixed IP address for your Raspberry Pi
10: Basic configuration
11: Working as the 'root' user
12: Raspberry Pi pins, roles, and numbers
13: A taste of Python on the Raspberry Pi
14: Python functions
15: A simple Python program
16: A simple circuit
17: Control an LED with GPIOZERO
18: Read a button with GPIOZERO
19: Setup the DHT22 sensor with Git
20: Use the DHT22 sensor
21: Raspberry Pi OS 64-bit vs 32-bit
We publish fresh content each week. Read how-to's on Arduino, ESP32, KiCad, Node-RED, drones and more. Listen to interviews. Learn about new tech with our comprehensive reviews. Get discount offers for our courses and books. Interact with our community. One email per week, no spam; unsubscribe at any time