RASPBERRY PI GETTING STARTED SERIES
Control An LED With GPIOZERO
You have an LED connected to GPIO4. How can you make it blink? I’ll show you in this lesson.
There are many ways to achieve the same result when it comes to programming and electronics. I will show you two ways to manipulate the LED.
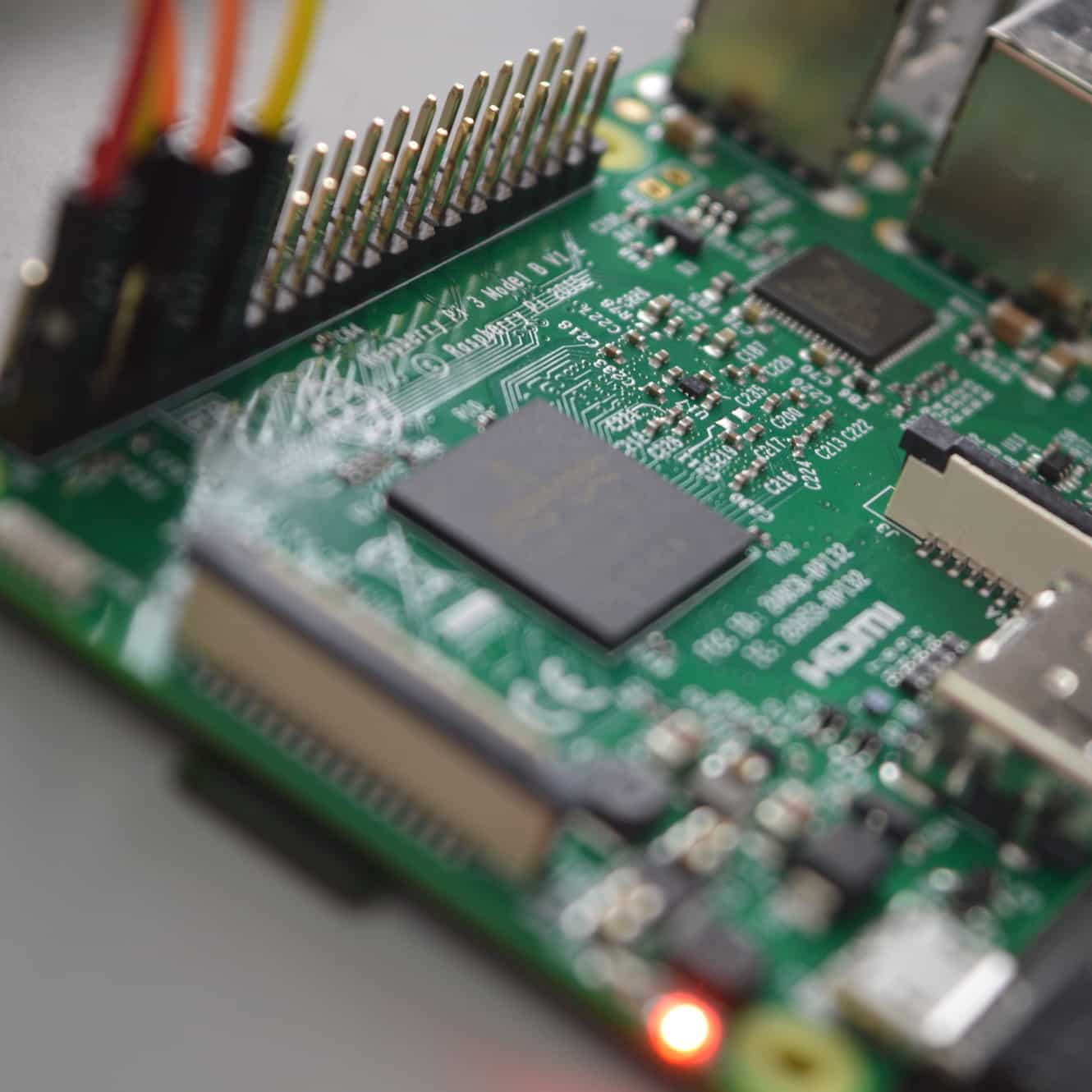
You have an LED connected to GPIO4. How can you make it blink? I’ll show you in this lesson.
As you can probably guess, there are many ways to achieve the same result when it comes to programming and electronics. I will show you two ways to manipulate the LED.
The first one involves using a feature of the GPIO Zero library that you installed in an earlier lesson. Back then, you only used this library to print out a pin map on the console. But the library contains a lot of functionality, including simple functions to manipulate an LED.
Let’s try it out.
Login to your Raspberry Pi as the “pi” user. Then, invoke the CLI for Python 3:
pi@raspberrypi-zero:~ $ python3
Python 3.7.3 (default, Dec 20 2019, 18:57:59)
[GCC 8.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>>
Next, import the LED module from the gpiozero library:
>>> from gpiozero import LED
This gives you access to the specific functions that allow you to manipulate the state of an LED.
Before you can turn the LED on, you must define the GPIO to which it is connected. Remember you have connected the anode of the LED to GPIO4.
So, you will issue this command:
>>> led = LED(4)
What you just did is to create an LED object that is connected to GPIO4 and assigned it to the “led” variable.
Now, you can call the “on()” and “off()” functions on this object to turn it on and off.
Try this:
>>> led.on()
Did your LED turn on? It should look like this:
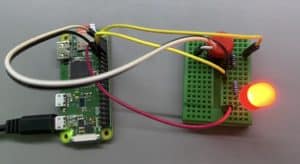
My LED, on.
Can you turn it off?
Try:
>>> led.off()
Here’s the complete CLI session:
pi@raspberrypi-zero:~ $ python3
Python 3.7.3 (default, Dec 20 2019, 18:57:59)
[GCC 8.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> from gpiozero import LED
>>> led = LED(4)
>>> led.on()
>>> led.off()
>>> exit()
You can also achieve the same thing by writing a small Python program and executing it with the Python3 interpreter.
Create a new text file with Vim, and call it “led_blink_gpiozero.py”. Type this code in the buffer:
from gpiozero import LED
from time import sleep
led = LED(4)
while True:
led.on()
sleep(1)
led.off()
sleep(1)
Your Vim should look like this:
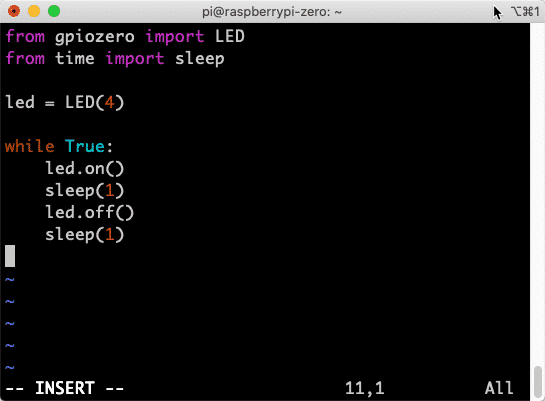
This program will blink an LED on and off in 1 second intervals.
Save your program and exit Vim (“:wq”).
Now, execute it like this:
$ python3 led_blink_gpiozero.py
Your LED will blink on and off.
In the code for this program, we imported the “time” Python module because it contains the “sleep” function. The “sleep” function is useful when you want to delay the execution of a program for a while. In our case, we used it to keep the LED on or off for 1 second. Feel free to replace “1” with another number if you want to create a different delay.
To end the program, use this keyboard shortcut: Ctr-C.
Ready for some serious learning?
Start right now with Raspberry Pi Full Stack - Raspbian
This is our most popular Raspberry Pi course & eBook.
This course is a hands-on project designed to teach you how to build an Internet-of-Things application based on the world’s most popular embedded computer.
You will learn how to build this application from the ground up, and gain experience and knowledge with technologies such as...
Jump to another article
1: What is the Raspberry Pi?
2: Raspberry Pi vs Arduino
3: Raspberry Pi operating systems
4: Headless and graphical (GUI) operating systems
5: How to install Raspbian Lite
6: SSH and headless configuration
7: How to set a host name
8: Booting for the first time
9: How to set a fixed IP address for your Raspberry Pi
10: Basic configuration
11: Working as the 'root' user
12: Raspberry Pi pins, roles, and numbers
13: A taste of Python on the Raspberry Pi
14: Python functions
15: A simple Python program
16: A simple circuit
17: Control an LED with GPIOZERO
18: Read a button with GPIOZERO
19: Setup the DHT22 sensor with Git
20: Use the DHT22 sensor
21: Raspberry Pi OS 64-bit vs 32-bit
We publish fresh content each week. Read how-to's on Arduino, ESP32, KiCad, Node-RED, drones and more. Listen to interviews. Learn about new tech with our comprehensive reviews. Get discount offers for our courses and books. Interact with our community. One email per week, no spam; unsubscribe at any time