RASPBERRY PI GETTING STARTED SERIES
Python Functions
A function is a block of code that does something useful. Think of functions as mini programs that are part of a larger program. They are an extremely useful programming building block, and, of course, you will use it a lot in this project.
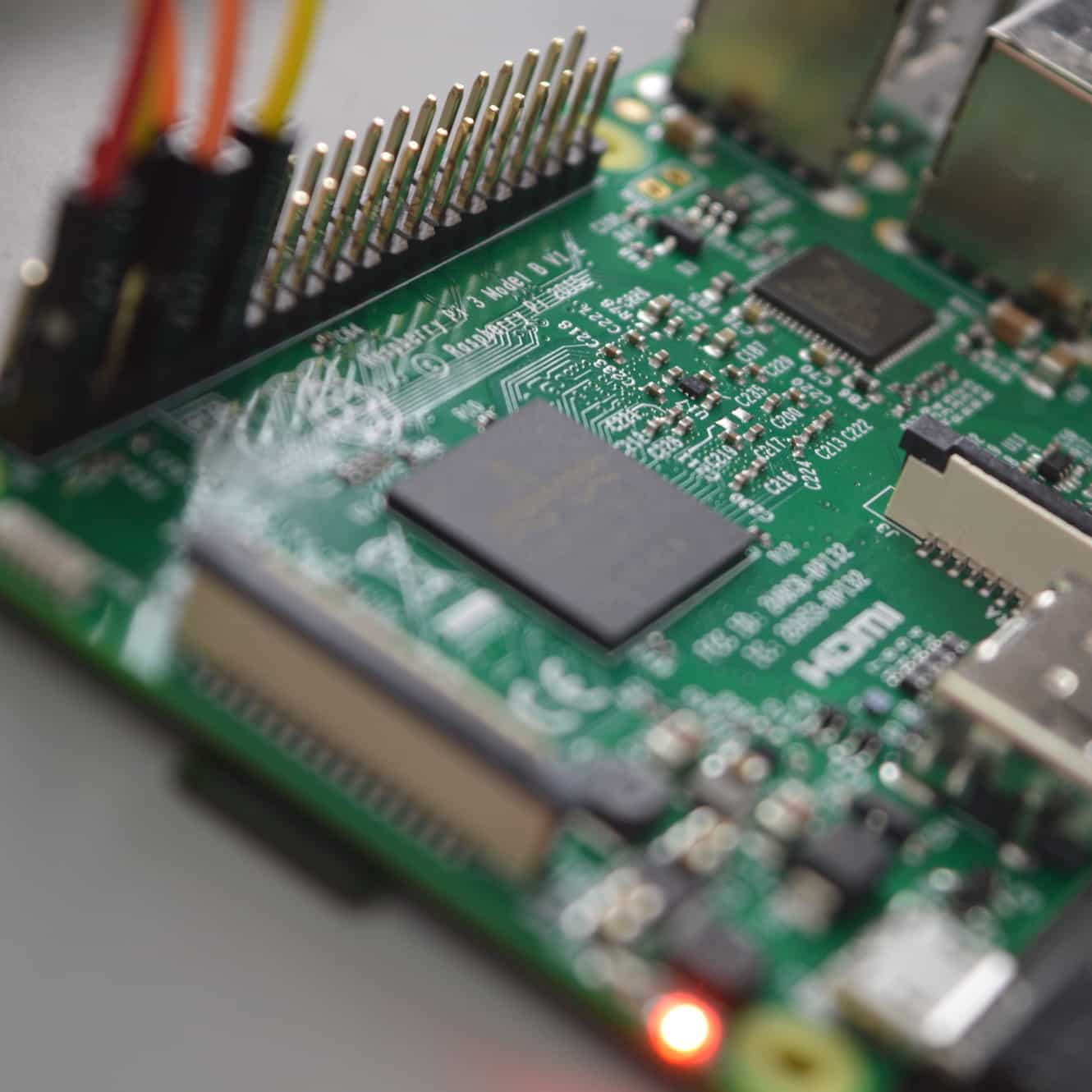
So, after the function definition, you have a line of code that multiplies the value stored in the “strn” variable by 3 and prints it to the console. Notice that the third line also begins with “…”. Python is not evaluating anything yet, it is waiting for your to give it the next instruction.
It is important to remember that white space is very important in Python. This is how Python can figure out which instruction belongs to a particular block. If you had not included the 3 spaces in the second line of this example, Python would assume that the new instruction does not belong to the function. These dependency on white space is a known pain point for Python programmers, and something that you will have to endure.
To keep this example super-simple, let end the function here, with only a single line of code. Your cursor is in line three. Hit Enter one more time. You should see this:
Noticed the “def” keyword, followed by the function name, the parameter in parentheses, and the “:” in the end that indicates the end of the function definition.
In the following lines you can write the code that makes up the function block. Let’s do this on the CLI:
Unless you are working on a trivial program, you will need a way to bundle multiple lines of code together. Modern programming languages have a handy way to do this: functions (also known as “methods”).
A function is a block of code that does something useful. Think of functions as mini programs that are part of a larger program. They are an extremely useful programming building block, and, of course, you will use it a lot in this project.
Since we are working with Python and the Command Line Interface, I’d like to show you how to create a simple function in this chapter.
Login to your Raspberry Pi as “pi”, and start the Python CLI:
$ python3
Now, let’s create a function. In Python, we use the keyword “def” to define a new function. A function definition needs a name (so we can use this name to call the function from somewhere else in our program), and an optional one or more attributes. An attribute can be a variety of things, like strings, numbers, variables, and arrays.
Here’s an example Python function, named “str_operation”, that has a single argument “strn”:
def str_operation(strn):
>>> def str_operation(strn):
...
The “…” in the CLI show that Python is aware that we are creating a function. It is waiting for us to write the function code, and is not evaluating anything until we “tell” it that our function is complete. So remember, when you see “…”, Python is waiting for you to type in an instruction that is part of the function.
Let’s continue to the second line. Press the space bar three times to move the start of the next instruction towards the right by three spaces, and type this in:
print(3 * strn)
Your CLI should look like this:
>>> def str_operation(strn):
... print(3 * strn)
...
>>> def str_operation(strn):
... print(3 * strn)
...
>>>
After the blank line, the CLI shows the familiar “>>>” prompt. Whatever you type now will be evaluated by python. Your new function exists in memory, and it will produce a result on the screen when you call it by name and pass an argument to it.
Now, try this:
>>> str_operation("Hello ")
You should get this back:
>>> str_operation("Hello ")
Hello Hello Hello
>>>
The string “Hello” has been copied three times in the console.
Nice!
You can modify this little function like this:
>>> def str_operation(strn):
... a = 3 * strn
... print(a)
...
>>>
This will evaluate the expression “3 * strn” and store the result in variable “a”, then print it to the console.
When you call it, the result is exactly the same as in the first version:
>>> str_operation("Hello ")
Hello Hello Hello
>>>
Yet another version of this example is the following:
>>> def str_operation(strn, num):
... a = num * strn
... print(a)
...
>>>
In this version, I have added a new parameter, called “num”. In the second line, I have replaced the original “3” with “num”, so that I can repeat the string stored in “strn” as many times as I like without having to modify the program.
I can call this function like this:
>>> str_operation("Hello ",4)
Hello Hello Hello Hello
>>>
Notice that inside the parentheses I have provided the string argument, and the number argument. In this call, the arguments appear in the same order as in the function definition; this is very important to remember as Python excepts the arguments to be in the order defined in the definition of the function.
As you can see, we use functions to bundle together instructions. We can call a function by its name, and pass any required parameters to it in order to have its instructions executed. But functions can also return values to the caller. Have a look at this variation of the ”str_operation” function:
def str_operation(strn, num):
return num * strn
In this example, the function definition is the same as in the original example. But, instead of the function doing the calculation and printing the result to the console, it simply returns the result of the calculation using the “return” keyword. The caller of the function can then decide what to do with the returned value.
Here is the example on the CLI:
>>> def str_operation(strn, num):
... return num * strn
...
>>> a = str_operation("Hello ", 3)
>>> print(a)
Hello Hello Hello
In this example, I have defined the new version of str_operation to return a value. Then, I call the function with its parameters, and store the returned value in a variable. Finally, I print the value stored in the “a” variable in the console.
There is a lot of flexibility in writing functions, using the return function, and in general how you structure your Python programs. For example, if you prefer a multi-step approach, you can write the function like this:
def str_operation(strn, num):
new_string = num * strn
return new_string
Here, your function does the calculation in one step, and the return of the value in another step.
Or, you can choose a more compact way of coding, like this:
def str_operation(strn, num):
return num * strn
print(str_operation("hello ", 3))
In this example, you have condensed four lines of code into 2 (the return line, and the print line), skipping the intermediate steps that involve the variables.
My personal preference is to code for clarity. I imagine other people reading my code, or myself reading my code sometime in the future. Will I (or my readers) be able to understand what my code does? Will they be able to improve it, or add new features? This means that usually it is bes to be more verbose, with sensible function and variable names, and a logical program structure.
From here, functions and programs get more elaborate and complicated, but you are already familiar with the basic principles.
Ready for something new? In the next chapter I will show you how to write a Python script that you can call from the command line, without using the CLI. You will build on the knowledge you gained in this chapter.
Ready for some serious learning?
Start right now with Raspberry Pi Full Stack - Raspbian
This is our most popular Raspberry Pi course & eBook.
This course is a hands-on project designed to teach you how to build an Internet-of-Things application based on the world’s most popular embedded computer.
You will learn how to build this application from the ground up, and gain experience and knowledge with technologies such as...
Jump to another article
1: What is the Raspberry Pi?
2: Raspberry Pi vs Arduino
3: Raspberry Pi operating systems
4: Headless and graphical (GUI) operating systems
5: How to install Raspbian Lite
6: SSH and headless configuration
7: How to set a host name
8: Booting for the first time
9: How to set a fixed IP address for your Raspberry Pi
10: Basic configuration
11: Working as the 'root' user
12: Raspberry Pi pins, roles, and numbers
13: A taste of Python on the Raspberry Pi
14: Python functions
15: A simple Python program
16: A simple circuit
17: Control an LED with GPIOZERO
18: Read a button with GPIOZERO
19: Setup the DHT22 sensor with Git
20: Use the DHT22 sensor
21: Raspberry Pi OS 64-bit vs 32-bit
We publish fresh content each week. Read how-to's on Arduino, ESP32, KiCad, Node-RED, drones and more. Listen to interviews. Learn about new tech with our comprehensive reviews. Get discount offers for our courses and books. Interact with our community. One email per week, no spam; unsubscribe at any time