RASPBERRY PI GETTING STARTED SERIES
Use The DHT22 Sensor
In this lesson you will take measurements from the DHT22 sensor that you wired in the previous lesson.
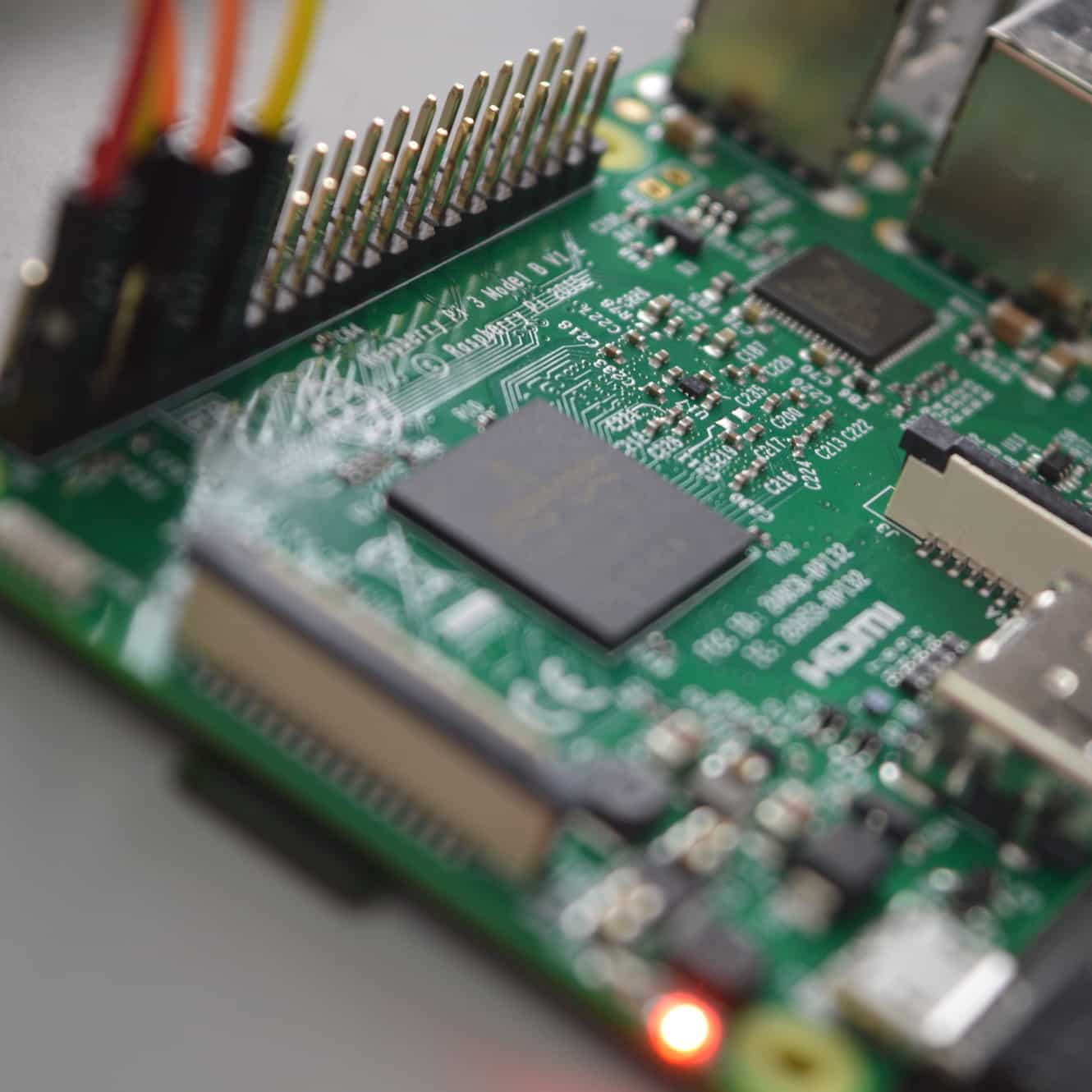
Let’s try out the DHT22 sensor with the Adafruit library.
From the root of your “pi” directory, change into the Adafruit DHT directory, and then into the examples directory:
$ cd Adafruit_Python_DHT/
$ cd examples/
Then, run the AdafruitDHT.py example, like this:
$ python3 AdafruitDHT.py 2302 17
Temp=23.6* Humidity=60.8%
Notice that there are two arguments that this example needs: the type of sensor you are using (“2302”) and the GPIO to which the sensor data pin is connected to (“17”). When the example program runs, you can an output with the temperature and humidity.
Now that you know that your sensor works, you can take a closer look at the example program to learn how it works. You can see inside any text file using the “cat” command, like this:
$ cat AdafruitDHT.py
I am copying the program here, but to economise on space I have removed most of the multi-line comments:
~/Adafruit_Python_DHT/examples $ cat AdafruitDHT.py
#!/usr/bin/python
# Copyright (c) 2014 Adafruit Industries
# Author: Tony DiCola
# … other comments
import sys
import Adafruit_DHT
# Parse command line parameters.
sensor_args = { '11': Adafruit_DHT.DHT11,
'22': Adafruit_DHT.DHT22,
'2302': Adafruit_DHT.AM2302 }
if len(sys.argv) == 3 and sys.argv[1] in sensor_args:
sensor = sensor_args[sys.argv[1]]
pin = sys.argv[2]
else:
print('Usage: sudo ./Adafruit_DHT.py [11|22|2302] <GPIO pin number>')
print('Example: sudo ./Adafruit_DHT.py 2302 4 - Read from an AM2302 connected to GPIO pin #4')
sys.exit(1)
# Try to grab a sensor reading. Use the read_retry method which will retry up
# to 15 times to get a sensor reading (waiting 2 seconds between each retry).
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin)
# Un-comment the line below to convert the temperature to Fahrenheit.
# temperature = temperature * 9/5.0 + 32
# Note that sometimes you won't get a reading and
# the results will be null (because Linux can't
# guarantee the timing of calls to read the sensor).
# If this happens try again!
if humidity is not None and temperature is not None:
print('Temp={0:0.1f}* Humidity={1:0.1f}%'.format(temperature, humidity))
else:
print('Failed to get reading. Try again!')
sys.exit(1)
The two import statements at the start of the program import the library itself (“Adafruit_DHT”), and the “sys” module. The “sys” (or “system”) module contains functions like “sys.argv”, used to get input from the command line, that you have seen in previous examples.
The “sensor_args“ list matches our input of the sensor model to the internal code for this model that the library understands. So, when we specify “2302”, the library internally will use “Adafruit_DHT.AM2302”.
Let’s jump to the interesting parts. This line:
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin)
… is where the program asks the sensor for humidity and temperature readings. We can get both readings in a single line of code. The result is a multiple assignment, where the humidity value is stored in the “humidity’ variable, and the temperature value is stored in the “temperature” variable.
The “read_retry” function needs two parameters: the type of sensor you are using, and the GPIO to which it is connected. Both of those values we specify in the command line when we invoke the program.
If you prefer the temperature in Fahrenheit, you can uncomment the conversion calculation just after the measurement is taken.
Another interesting segment in this program is this:
if humidity is not None and temperature is not None:
print('Temp={0:0.1f}* Humidity={1:0.1f}%'.format(temperature, humidity))
else:
print('Failed to get reading. Try again!')
sys.exit(1)
This is where the program tests for the validity of the result from the sensor. If the result is a number (instead of “None”), then it will print out the results. Otherwise it will print out a “failed” message.
Behind the scenes, a fairly sophisticated choreography of actions too place. But from our perspective, and end users of the sensor module and the library, we accomplished what we wanted with a single line of code. This is an example of the power of Python and its library ecosystem.
Rapid prototyping.
Ready for some serious learning?
Start right now with Raspberry Pi Full Stack - Raspbian
This is our most popular Raspberry Pi course & eBook.
This course is a hands-on project designed to teach you how to build an Internet-of-Things application based on the world’s most popular embedded computer.
You will learn how to build this application from the ground up, and gain experience and knowledge with technologies such as...
Jump to another article
1: What is the Raspberry Pi?
2: Raspberry Pi vs Arduino
3: Raspberry Pi operating systems
4: Headless and graphical (GUI) operating systems
5: How to install Raspbian Lite
6: SSH and headless configuration
7: How to set a host name
8: Booting for the first time
9: How to set a fixed IP address for your Raspberry Pi
10: Basic configuration
11: Working as the 'root' user
12: Raspberry Pi pins, roles, and numbers
13: A taste of Python on the Raspberry Pi
14: Python functions
15: A simple Python program
16: A simple circuit
17: Control an LED with GPIOZERO
18: Read a button with GPIOZERO
19: Setup the DHT22 sensor with Git
20: Use the DHT22 sensor
21: Raspberry Pi OS 64-bit vs 32-bit
Last Updated 1 year ago.
We publish fresh content each week. Read how-to's on Arduino, ESP32, KiCad, Node-RED, drones and more. Listen to interviews. Learn about new tech with our comprehensive reviews. Get discount offers for our courses and books. Interact with our community. One email per week, no spam; unsubscribe at any time